WPFでボタンを配置
XAML上ではこんな感じです
<Window x:Class="ReadText.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:ReadText"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid>
<Button x:Name="B_readCSV" Content="CSV読込" HorizontalAlignment="Left" VerticalAlignment="Top" Width="147" Click="B_readCSV_Click" Height="52"/>
</Grid>
</Window>
B_readCSV ボタンにクリックイベントを実装しました
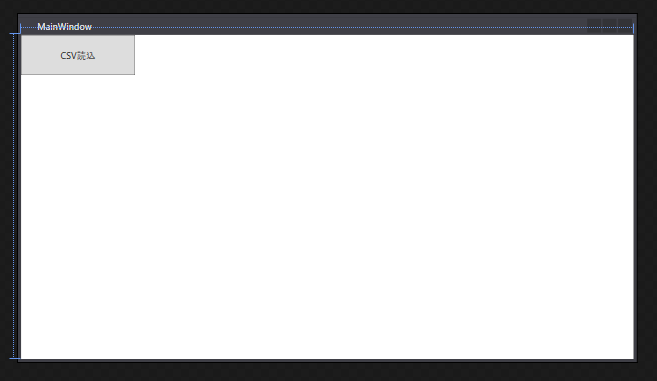
ファイルを開く処理 OpenFileDialog
usingを使うとusingで指定した名前空間に属するクラスや構造体などを使用する際に、名前空間を省いてコードを書くことができます。
using Microsoft.Win32;
OpenFileDialogを利用してファイルを開く
private void B_readCSV_Click(object sender, RoutedEventArgs e)
{
//OpenFileDialogオブジェクト作成
var ofd = new OpenFileDialog();
//拡張子を指定
ofd.Filter = "CSVファイル(*.csv)|*.csv";
//ダイアログの処理です。
if (ofd.ShowDialog() == true)
{
MessageBox.Show(ofd.FileName);
}
}
開くダイアログが表示されます。
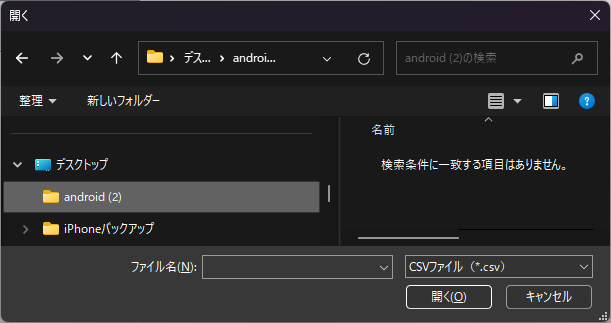
ofd.FileNameにはファイルまでのパスが代入されます
StreamReaderで読み込む
OpenFileDialogで取得したファイルパスを用いて、テキストファイル内にあるテキストを1行ずつ読込、LISTへ格納していきます。
最終的に1行ずつ、メッセージボックスにて表示します。
private void B_readCSV_Click(object sender, RoutedEventArgs e)
{
//OpenFileDialogのオブジェクトを作成します。
var ofd = new OpenFileDialog();
//ファイルの拡張子指定を行います。
ofd.Filter = "CSVファイル(*.csv)|*.csv";
//ダイアログの処理です。
if (ofd.ShowDialog() == true)
{
//ファイルパスを表示する
MessageBox.Show(ofd.FileName);
//読み込むテキストを保存する変数
var texts = new List<string>();
try
{
//ファイルをオープンする
using (StreamReader sr = new StreamReader(ofd.FileName, Encoding.GetEncoding("Shift_JIS")))
{
while (0 <= sr.Peek())
{
texts.Add(sr.ReadLine());
}
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
//読み込んだテキストを1行ずつ表示する
foreach (var text in texts) { MessageBox.Show(text); }
}
}
コメント